IndexedDB
Service Workers, and Background Sync API, you can create an offline friendly image upload system that uploads and automatically try again-so that your user can upload stress-free, even offline.
Therefore, you are filling an online form, and it asks you to upload the file. You click on the input, select the file from your desktop, and are good at going. But something happens. The network drops, the file disappears, and you get stuck to upload the file. Poor Network Connectivity You can lead to unreasonable time trying to upload files successfully.
What is ruined the user experience is created by permanently checking the stability of the network and repeating the upload several times. Although we may not be able to work more about network contact, as a developer, we can always do something to reduce the pain that comes with this problem.
One of the ways to solve this problem is to tweet the image upload system in a way that enables users to upload offline images. Eliminating the need for reliable network connectionAnd then, when the network becomes stable without the consumer’s intervention, try the system upload process again.
Is going to focus on explaining this article how to build An offline friendly image upload system PWA (progressive web application) use technologies such as IndexedDB
Service worker, and background synchronization API. We will also briefly cover the points to improve the user experience for this system.
Planning offline image upload system
Here is a flu chart for offline friendly image upload system.
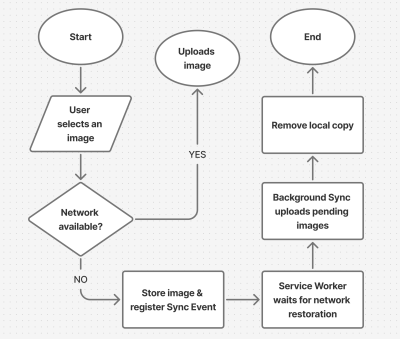
As shown in the flu chart, the process is as follows:
- The user selects a picture.
This process begins by allowing the user to select his image. - The syllable is locally secure
IndexedDB
.
Next, the system checks for network contact. If network contact is available, the system uploads the system directly, avoiding the use of unnecessary local storage. However, if the network is not available, the image will be stored inIndexedDB
. - When the network is restored, the service worker detects.
With storing in the pictureIndexedDB
The network connection is restored to continue with the system, when the next step is to be detected. - The process of background synchronization is pending upload.
When the connection is restored, the system will try to upload the image again. - The file is uploaded with success.
When the icon is uploaded, the local copy stored in the system will be removedIndexedDB
.
Implement the system
The first step in the implementation of the system allows the user to select his photos. There are different ways you can get it:
I would advise you to use the two. Some users prefer to use the drag and drop interface, while others think the only way to upload images is through the Lord Placing both options will help improve the user’s experience. You may also consider allowing users to paste photos directly into the browser using the Clipboard API.
Registering service worker
This solution is a service worker in the heart. Our service worker will be responsible for recovering this photo from this photo IndexedDB
Upload it when internet connection is restored, and clean it IndexedDB
Store when the photo is uploaded.
You have to use the service worker, you must first register:
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js')
.then(reg => console.log('Service Worker registered', reg))
.catch(err => console.error('Service Worker registration failed', err));
}
Checking network contacts
Remember, the problem we are trying to solve is because Incredible network connectivity. If this problem does not exist, there is no point in trying to solve anything. So, once the icon is selected, we need to check if the user has no reliable internet connection before registering the sync event and storing the image IndexedDB
.
function uploadImage() {
if (navigator.onLine) {
// Upload Image
} else {
// register Sync Event
// Store Images in IndexedDB
}
}
Note: I’m just using navigator.onLine
Here property to show how the system will work. navigator.onLine
Is the property IncredibleAnd I will advise you to come up with a custom solution to find out if the user is connected to the Internet. One way you can do this is that you have sent a ping request at the end of the server’s closing point.
Enrollment of the synchronous event
Once the network fails the test, the next step is to register the synchronization program. The sync event needs to be registered at a location where the system fails to upload the image due to poor internet connection.
async function registerSyncEvent() {
if ('SyncManager' in window) {
const registration = await navigator.serviceWorker.ready;
await registration.sync.register('uploadImages');
console.log('Background Sync registered');
}
}
After registering the synchronous event, you need to hear it in the service worker.
self.addEventListener('sync', (event) => {
if (event.tag === 'uploadImages') {
event.waitUntil(sendImages());
}
});
sendImages
Function is going to be a contradictory process that will recover this photo IndexedDB
And upload it to the server. It is just like:
async function sendImages() {
try {
// await image retrieval and upload
} catch (error) {
// throw error
}
}
Open the database
We need to work first to store our icons locally IndexedDB
Store as you can see from the code below, we are creating A global variable to store the database example. The reason to do this is, after that, when we want to recover our image IndexedDB
We will not need to write a code to open the database again.
let database; // Global variable to store the database instance
function openDatabase() {
return new Promise((resolve, reject) => {
if (database) return resolve(database); // Return existing database instance
const request = indexedDB.open("myDatabase", 1);
request.onerror = (event) => {
console.error("Database error:", event.target.error);
reject(event.target.error); // Reject the promise on error
};
request.onupgradeneeded = (event) => {
const db = event.target.result;
// Create the "images" object store if it doesn't exist.
if (!db.objectStoreNames.contains("images")) {
db.createObjectStore("images", { keyPath: "id" });
}
console.log("Database setup complete.");
};
request.onsuccess = (event) => {
database = event.target.result; // Store the database instance globally
resolve(database); // Resolve the promise with the database instance
};
});
}
To store photo in Indexed DB
With IndexedDB
Open store, now we can store our photos.
Now, you’ll be wondering why a simple solution is like
localStorage
Was not used for this purpose.The reason for this is
IndexedDB
Runs inadvertently and does not block the main thread of JavaScript, whilelocalStorage
It runs in harmony and can block if the main thread of the JavaScript is being used.
Here’s how you can store the picture IndexedDB
:
async function storeImages(file) {
// Open the IndexedDB database.
const db = await openDatabase();
// Create a transaction with read and write access.
const transaction = db.transaction("images", "readwrite");
// Access the "images" object store.
const store = transaction.objectStore("images");
// Define the image record to be stored.
const imageRecord = {
id: IMAGE_ID, // a unique ID
image: file // Store the image file (Blob)
};
// Add the image record to the store.
const addRequest = store.add(imageRecord);
// Handle successful addition.
addRequest.onsuccess = () => console.log("Image added successfully!");
// Handle errors during insertion.
addRequest.onerror = (e) => console.error("Error storing image:", e.target.error);
}
With stored images and background sync sets, the system is ready to upload the image whenever a network connection is restored.
Recover and upload photos
Once the network connection is restored, the synchronous incident will be fired, and the service worker will recover this photo IndexedDB
And upload it.
async function retrieveAndUploadImage(IMAGE_ID) {
try {
const db = await openDatabase(); // Ensure the database is open
const transaction = db.transaction("images", "readonly");
const store = transaction.objectStore("images");
const request = store.get(IMAGE_ID);
request.onsuccess = function (event) {
const image = event.target.result;
if (image) {
// upload Image to server here
} else {
console.log("No image found with ID:", IMAGE_ID);
}
};
request.onerror = () => {
console.error("Error retrieving image.");
};
} catch (error) {
console.error("Failed to open database:", error);
}
}
Deleting Indexeddb Database
Once the syllable has been uploaded, IndexedDB
No need for a store anymore. Therefore, it should be deleted with its content to free the storage.
function deleteDatabase() {
// Check if there's an open connection to the database.
if (database) {
database.close(); // Close the database connection
console.log("Database connection closed.");
}
// Request to delete the database named "myDatabase".
const deleteRequest = indexedDB.deleteDatabase("myDatabase");
// Handle successful deletion of the database.
deleteRequest.onsuccess = function () {
console.log("Database deleted successfully!");
};
// Handle errors that occur during the deletion process.
deleteRequest.onerror = function (event) {
console.error("Error deleting database:", event.target.error);
};
// Handle cases where the deletion is blocked (e.g., if there are still open connections).
deleteRequest.onblocked = function () {
console.warn("Database deletion blocked. Close open connections and try again.");
};
}
With this, the whole process is complete!
Reservations and limits
Although we have done a lot to help improve the experience by supporting offline uploads, this system is not without its limits. I thought I would especially call them because it is worth noting that this solution may be less than your needs.
- No reliable internet contact addresses
Javascript does not provide a foolproof method for online status. For this reason, you need to come up with a custom solution to detect online status. - Only chromium solution
Background sync is currently API Limited to chromium -based browsers. Thus, this solution is supported only by chromium browsers. This means that if you have the majority of your customers on non -chromium browsers, you will need a strong solution. IndexedDB
Storage policies
Browser storage limits and evacuation policiesIndexedDB
. For example, in Safari, is stored in dataIndexedDB
If the user does not interact with the website, it is seven days old. If you come with a background synchronous API alternative that supports Safari, you should keep in mind.
Increase the user experience
Since this is the whole process in the background, we need a way to inform users when the images are stored, awaits uploads, or has been successfully uploaded. To enforce something Ui elements For this purpose, consumer experience will really increase. These UI elements include toast notifications, spinners (to display active actions), development bars (to show state progress), network status indicators, or buttocks, indicators of the Progress Status, upload status, upload status.
Wrap
Poor Internet Connectivity can disrupt the user’s experience of a web application. However, by taking advantage of PWA Technologies IndexedDB
Service workers, and background sync API, developers can help their users improve the reliability of web applications, especially in areas that are incredible Internet contacts.

(GG, Wii’s)
Unlock Your Business Potential with Stan Jackowski Designs
At Stan Jackowski Designs, we bring your ideas to life with cutting-edge creativity and innovation. Whether you need a customized website, professional digital marketing strategies, or expert SEO services, we’ve got you covered! Our team ensures your business, ministry, or brand stands out with high-performing solutions tailored to your needs.
🚀 What We Offer:
- Web Development – High-converting, responsive, and optimized websites
- Stunning Design & UI/UX – Eye-catching visuals that enhance engagement
- Digital Marketing – Creative campaigns to boost your brand presence
- SEO Optimization – Increase visibility, traffic, and search rankings
- Ongoing Support – 24/7 assistance to keep your website running smoothly
🔹 Take your business to the next level! Explore our outstanding services today:
Stan Jackowski Services
📍 Located: South of Chicago
📞 Contact Us: https://www.stanjackowski.com/contact/
💡 Bonus: If you’re a ministry, church, or non-profit organization, we offer specialized solutions, including website setup, training, and consultation to empower your online presence. Book a FREE 1-hour consultation with Rev. Stanley F. Jackowski today!
🔥 Looking for a done-for-you autoblog website? We specialize in creating money-making autoblog websites that generate passive income on autopilot. Let us handle the technical details while you focus on growth!
📩 Let’s Build Something Amazing Together! Contact us now to get started.